TOC
Asp .Net Core 3.x
Asp .Net Core
本质是 一个Server
+ 多个中间件(Middleware)组成的管道(Pipline)
。
本身是一个console application
,经 Main() > CreateHostBuilder(args).Build()
后成为web application
。
Asp .Net Core 应用多样性
- MVC:/Home/Index
- Razor Pages: /SomePage
- SignalR: /Hub/Chat
2. Razor Page
MVC
- Model: 数据
- View: Html, Razor, TagHelpers
- Controller: 逻辑
Razor Page
- 数据
- Html, Razor, TagHelpers
- 逻辑
Create Project
- New > Project > Asp .Net core web application
Startup.cs
public class Startup
{
public void ConfigureServices(IServiceCollection services)
{
services.AddRazorPages();
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
app.UseStaticFiles();
app.UseHttpsRedirection();
app.UseAuthentication();
app.UseRouting();
app.UseEndpoints(endpoints =>
{
endpoints.MapRazorPages();
});
}
}
Create Models, Service and Register services
-
Startup.cs
public void ConfigureServices(IServiceCollection services) { services.AddRazorPages(); services.AddSingleton<IDepartmentService, DepartmentService>(); services.AddSingleton<IEmployeeService, EmpolyeeService>(); }
Config配置信息源
-
appsettings.json
{ "Logging": { "LogLevel": { "Default": "Information", "Microsoft": "Warning", "Microsoft.Hosting.Lifetime": "Information" } }, "AllowedHosts": "*", "AspCoreRazorDemo": { "BoldDepartmentEmployeeC: 30 } }
Startup.cs 注入Config,映射为options类
private readonly IConfiguration configuration;
public Startup(IConfiguration configuration)
{
this.configuration = configuration;
}
public void ConfigureServices(IServiceCollection services)
{
services.AddRazorPages();
services.AddSingleton<IDepartmentService, DepartmentService>();
services.AddSingleton<IEmployeeService, EmpolyeeService>();
services.Configure<AspCoreRazorDemoOptions>(configuration.GetSection("AspCoreRazorDemo"));
}
Using libman Install bootstrap
-
Add > Client-Side Library > bootstrap@4.4.1 > Install, libman.json
{ "version": "1.0", "defaultProvider": "unpkg", "libraries": [ { "library": "bootstrap@4.4.1", "destination": "wwwroot/lib/bootstrap/", "files": [ "dist/css/bootstrap.css" ] } ] }
Using BuildBundlerMinifier Merge and Minify css files
-
Add > New Item, bundleconfig.json
[ { "outputFileName": "wwwroot/css/all.min.css", "inputFiles": [ "wwwroot/css/site.css", "wwwroot/lib/bootstrap/dist/css/bootstrap.css" ] }, { "outputFileName": "wwwroot/css/bootstrap.css", "inputFiles": [ "wwwroot/lib/bootstrap/dist/css/bootstrap.css" ], "minify": {"enabled": true} } ]
-
Manage NuGet packges > BuildBundlerMinifier
ASPNETCORE_ENVIRONMENT
-
\Properties\launchSettings.json
{ "profiles": { "AspCoreRazorDemo": { "commandName": "Project", "launchBrowser": true, "applicationUrl": "https://localhost:5001;http://localhost:5000", "environmentVariables": { "ASPNETCORE_ENVIRONMENT": "Development" } } } }
Views
-
Views > _ViewImports.cshtml 全局启用TagHelper : Add > New Item > Razor View Imports
@using AspCoreRazorDemo @namespace AspCoreRazorDemo.Pages @addTagHelper "*, Microsoft.AspNetCore.Mvc.TagHelpers"
-
Views > Shared > _Layout.cshtml 公共页面 : Add > New Item > Razor Layout
<!DOCTYPE html> <html> <head> <meta name="viewport" content="width=device-width" /> <title>@ViewBag.Title</title> <environment include="Development"> <link rel="stylesheet" asp-href-include="css/*" asp-href-exclude="css/all.main.css" /> </environment> <environment exclude="Development"> <link rel="stylesheet" asp-href-include="css/all.main.css" /> </environment> </head> <body> <div class="container"> <div class="row"> <div class="col-md-2"> <!-- taghelper: asp-append-version 防止图片被缓存 --> <img asp-append-version="true" alt="logo" src="~/images/Home.png" style="height: 60px; width: 100px;" /> </div> <div class="col-md-10"> <span class="h2">@ViewBag.Title</span> </div> </div> <div class="row"> <div class="col-md-12"> @RenderBody() </div> </div> </div> </body> </html>
-
Views > _ViewStart.cshtml start 页面: Add > New Item > Razor View Start
@{ Layout = "_Layout"; }
-
Pages > Index.cshtml : Add > NewItem > Razor View
@page @using AspCoreRazorDemo.Models @using AspCoreRazorDemo.Services @inject IDepartmentService departmentService <div class="row"> <div class="col-md-10 offset-md-2"> <table class="table"> <tr> <th>Name</th> <th>Location</th> <th>EmployeeCount</th> <th>Opration</th> </tr> @Html.DisplayFor(x => x.Departments) </table> </div> </div> <div class="row"> <div class="col-md-4"> <a asp-page="Department/AddDepartment">Add</a> </div> </div> @functions { public IEnumerable<Department> Departments { get; set; } public async Task OnGetAsync() { Departments = await departmentService.GetAll(); } }
-
Pages > DisplayTemplates > Department.cshtml: Add > NewItem > Razor View
@using AspCoreRazorDemo.Models @using Microsoft.Extensions.Options @model AspCoreRazorDemo.Models.Department @inject IOptions<AspCoreRazorDemoOptions> options <tr> @if (Model.EmployeeCount > options.Value.BoldDepartmentEmployeeCount) { <td><strong>@Model.Name</strong></td> } else { <td>@Model.Name</td> } <td>@Model.Location</td> <td>@Model.EmployeeCount</td> </tr>
-
Pages > Department> AddDepartment.cshtml: Add > New Item > Razor Page
@page @model AspCoreRazorDemo.Pages.Department.AddDepartmentModel <form method="post"> <div class="row form-group"> <div class="col-md-2 offset-md-2"> <label asp-for="Department.Name"></label> </div> <div class="col-md-6"> <input class="form-control" asp-for="Department.Name" /> </div> </div> <div class="row form-group"> <div class="col-md-2 offset-md-2"> <label asp-for="Department.Location"></label> </div> <div class="col-md-6"> <input class="form-control" asp-for="Department.Location" /> </div> </div> <div class="row form-group"> <div class="col-md-2 offset-md-2"> <label asp-for="Department.EmployeeCount"></label> </div> <div class="col-md-6"> <input class="form-control" asp-for="Department.EmployeeCount" /> </div> </div> <div class="row"> <div class="col-md-2 offset-md-2"> <button type="submit" class="btn btn-primary">Add</button> </div> </div> </form>
-
Pages > Department> AddDepartment.cshtml.cs
public class AddDepartmentModel : PageModel { private readonly IDepartmentService departmentService; [BindProperty] public AspCoreRazorDemo.Models.Department Department { get; set; } public AddDepartmentModel(IDepartmentService departmentService) { this.departmentService = departmentService; } public async Task<IActionResult> OnPostAsync() { if (ModelState.IsValid) { await departmentService.Add(Department); return RedirectToPage("/Index"); } return Page(); } }
ViewComponet 可重用组件
-
Pages> Shared> Components> CompanySummary> Default.cshtml
-
ViewComponents > CompanySummaryViewComponent.cs
-
Views\_ViewImports.cshtml 引入本项目程序集
@using AspCoreRazorDemo @namespace AspCoreRazorDemo.Pages @addTagHelper "*, Microsoft.AspNetCore.Mvc.TagHelpers" @addTagHelper "*, AspCoreRazorDemo"
-
Pages\Index.cshtml 使用ViewComponent
<div class="row"> <div class="col-md-2"> @await Component.InvokeAsync("CompanySummary", new { title = "Summary of Company" }) <vc:company-summary title="Summary"></vc:company-summary> </div> <div class="col-md-4"> <a asp-page="Department/AddDepartment">Add</a> </div> </div>
项目地址
github: https://github.com/leezhuang96/Asp.NetCoreDemo
「下次一定」
下次一定
使用微信扫描二维码完成支付
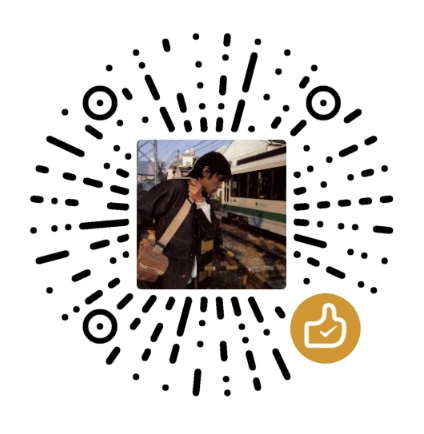