TOC
ECMAScript 6 简介
ECMAScript 6.0(以下简称 ES6)是 JavaScript 语言的下一代标准,已经在 2015 年 6 月正式发布了。它的目标,是使得 JavaScript 语言可以用来编写复杂的大型应用程序,成为企业级开发语言。
Class 的基本语法
Class的概念
- 基本用法:
JavaScript
语言中,生成实例对象的传统方法是通过构造函数。ES6 提供了更接近传统语言的写法,引入了Class
(类)这个概念,作为对象的模板。通过class
关键字,可以定义类。基本上,ES6 的class
可以看作只是一个语法糖,新的class
写法只是让对象原型的写法更加清晰、更像面向对象编程的语法而已//ES5: function Point(x, y) { this.x = x; this.y = y; } Point.prototype.toString = function () { return '(' + this.x + ', ' + this.y + ')'; }; var p = new Point(1, 2); //ES6: class Point { constructor(x, y) { this.x = x; this.y = y; } toString() { return '(' + this.x + ', ' + this.y + ')'; } }
constructor 方法
- 基本用法:
constructor
方法是类的默认方法,通过new命令生成对象实例时,自动调用该方法。一个类必须有constructor
方法,如果没有显式定义,一个空的constructor
方法会被默认添加。class Point { } // 等同于 class Point { constructor() {} }
实例属性
- 基本用法:
实例属性除了定义在
constructor()
方法里面的this
上面,也可以定义在类的最顶层。class IncreasingCounter { constructor() { this._count = 0; } get value() { console.log('Getting the current value!'); return this._count; } increment() { this._count++; } } //or class IncreasingCounter { _count = 0; get value() { console.log('Getting the current value!'); return this._count; } increment() { this._count++; } }
取值函数(getter)和存值函数(setter)
- 基本用法:
与 ES5 一样,在“类”的内部可以使用
get
和set
关键字,对某个属性设置存值函数和取值函数,拦截该属性的存取行为。class MyClass { constructor() { // ... } get prop() { return 'getter'; } set prop(value) { console.log('setter: '+value); } } let inst = new MyClass(); inst.prop = 123; // setter: 123 inst.prop // 'getter'
注意点
- 严格模式: 类和模块的内部,默认就是严格模式,所以不需要使用
use strict
指定运行模式 Generator
方法: 如果某个方法之前加上星号(*
),就表示该方法是一个Generator
函数this
的指向: 类的方法内部如果含有this
,它默认指向类的实例
静态方法
- 基本用法:
类相当于实例的原型,所有在类中定义的方法,都会被实例继承。如果在一个方法前,加上
static
关键字,就表示该方法不会被实例继承,而是直接通过类来调用,这就称为静态方法
。class Foo { static classMethod() { return 'hello'; } } Foo.classMethod() // 'hello' var foo = new Foo(); foo.classMethod() // TypeError: foo.classMethod is not a function //如果静态方法包含this关键字,这个this指的是类,而不是实例。 class Foo { static bar() { this.baz(); } static baz() { console.log('hello'); } baz() { console.log('world'); } } Foo.bar() // hello
new.target 属性
- 基本用法:
ES6 为
new
命令引入了一个new.target
属性,该属性一般用在构造函数之中,返回new
命令作用于的那个构造函数。如果构造函数不是通过new
命令或Reflect.construct()
调用的,new.target
会返回undefined
,因此这个属性可以用来确定构造函数是怎么调用的。function Person(name) { if (new.target === Person) { this.name = name; } else { throw new Error('必须使用 new 命令生成实例'); } } var person = new Person('张三'); // 正确 var notAPerson = Person.call(person, '张三'); // 报错
Class
内部调用new.target
,返回当前Class
。class Rectangle { constructor(length, width) { console.log(new.target === Rectangle); this.length = length; this.width = width; } } var obj = new Rectangle(3, 4); // 输出 true
子类继承父类时,
new.target
会返回子类。class Rectangle { constructor(length, width) { console.log(new.target === Rectangle); // ... } } class Square extends Rectangle { constructor(length) { super(length, width); } } var obj = new Square(3); // 输出 false
Class 的继承
继承的概念
- 基本用法:
Class
可以通过extends
关键字实现继承,这比 ES5 的通过修改原型链实现继承,要清晰和方便很多。子类必须在constructor
方法中调用super
方法,否则新建实例时会报错。class Point { ... } class ColorPoint extends Point { constructor(x, y, color) { super(x, y); // 调用父类的constructor(x, y) this.color = color; } toString() { return this.color + ' ' + super.toString(); // 调用父类的toString() } }
- 差异:
- ES5 的继承:实质是先创造子类的实例对象
this
,然后再将父类的方法添加到this
上面(Parent.apply(this)
)。 - ES6 的继承机制完全不同: 实质是先将父类实例对象的属性和方法,加到
this
上面(所以必须先调用super
方法),然后再用子类的构造函数修改this
。
- ES5 的继承:实质是先创造子类的实例对象
Object.getPrototypeOf()
- 基本用法:
Object.getPrototypeOf
方法可以用来从子类上获取父类。因此,可以使用这个方法判断,一个类是否继承了另一个类。Object.getPrototypeOf(ColorPoint) === Point // true
super 关键字
- 基本用法:
super
这个关键字,既可以当作函数使用,也可以当作对象使用。super
作为函数调用时: 代表父类的构造函数。ES6 要求,子类的构造函数必须执行一次super
函数,super
内部的this
指的是子类的实例。作为函数时,super()
只能用在子类的构造函数之中,用在其他地方就会报错。super
作为对象时: 在普通方法中,指向父类的原型对象;在静态方法中,指向父类。ES6 规定,在子类普通方法中通过super
调用父类的方法时,方法内部的this
指向当前的子类实例。
类的 prototype 属性和__proto__属性
- 基本用法:
- 子类的
__proto__
属性,表示构造函数的继承,总是指向父类。 - 子类
prototype
属性的__proto__
属性,表示方法的继承,总是指向父类的prototype
属性。
class A { } class B extends A { } B.__proto__ === A // true B.prototype.__proto__ === A.prototype // true
- 子类的
实例的 proto 属性
- 基本用法:
子类实例的
__proto__
属性的__proto__
属性,指向父类实例的__proto__
属性。也就是说,子类的原型的原型,是父类的原型。class A { } class B extends A { } var a = new A(); var b = new B(); b.__proto__ === a.__proto__ // false b.__proto__.__proto__ === a.__proto__ // true
原生构造函数的继承
- 基本用法: 原生构造函数是指语言内置的构造函数,通常用来生成数据结构。以前,这些原生构造函数是无法继承的,ES6 允许继承原生构造函数定义子类。
「下次一定」
下次一定
使用微信扫描二维码完成支付
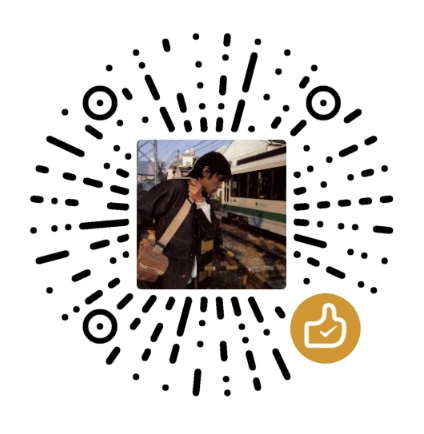